Saturday, January 5, 2013
SQL Limit in PHP
Introduction
PHP
has an operator named LIMIT. Using the LIMIT operator you can fetch a
minimum and maximum row from a database. In this article you will see,
how to execute the LIMIT operator of MySQL in PHP.
Suppose
that there are ten rows in a table of any database in MySQL. You want
to fetch only five rows from your database; then you will use the LIMIT operator.
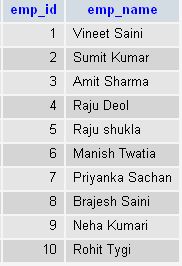
Suppose there is the above table in your database. You want to fetch only five rows from this table. For this purpose you will use the LIMIT operator. An example of the LIMIT operator is as follows:
Example 1. If you want to see only five rows from a table in MySQL then you will use the following query:
select * from emp limit 5
Output
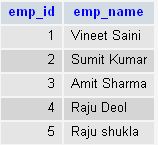
Example 2. If you want to see only five rows from a table in PHP form, then you will use following code.
In this example, we have created a limit.php page, in which we execute a query with the LIMIT operator. In the LIMIT operator we put minimum and maximum limit of the table data. For example, the LIMIT 0,5 retrieves the row starting from row number 0 (the first one) to a maximum of 5 rows. Code
<title>Limit in PHP</title>
<body bgcolor="pink">< h3><u>SQL Limit in PHP<u></h3>
<center> $con = mysql_connect("localhost","root","");
if (!$con) {
die( 'Could not connect: ' . mysql_error());
} mysql_select_db ("sunderdeep", $con); // sunderdeep is a database name $result = mysql_query ("select * from emp limit 0,5"); // emp is the table name in database echo "
emp_id | emp_Name |
---|
while ($row = mysql_fetch_array ($result)) {
echo "
echo "
echo "
echo "";
}echo "";
mysql_close ($con);?></center>
</body>
</body>
The above file is saved with the name limit.php.
Output
When you browse your limit.php file then you will get the following output:
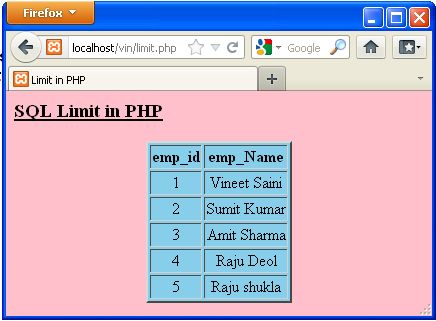
Conclusion
So
in this article you saw, how to execute the LIMIT operator of MySQL in
PHP. Using this article one can easily understand how to execute the
LIMIT operator of MySQL in PHP.
Delete Statement in PHP
In the last article I explained how to update and select data from a MySql database into a HTML table. In this article I will explain how to delete data in the database through HTML table. In this article you will see, data deleted from the front end i.e. HTML table.
Code
$con=mysql_connect ("localhost","root","");
mysql_select_db ("mcn",$con);
@$a=$_POST['txt1'];
@$b=$_POST['txt2'];
@$c=$_POST['txt3'];
@$d=$_POST['txt4'];if(@$_POST ['del'])
{
$s="delete from employee where name='$a'";echo "Your Data Deleted";
mysql_query ($s);
}
$con=mysql_connect ("localhost","root","");
mysql_select_db ("mcn",$con);if(@$_POST ['sel'])
{echo $ins=mysql_query ("select * from employee");echo "
Select Data From Employee Table | |||
---|---|---|---|
Nmae | Designation | Sal | Qualification |
while ($row=mysql_fetch_array($ins))
{
echo "
echo "
echo "
echo "
echo "
echo "";
}
}
echo ""
?><html>
<head>
</head>
<body bgcolor="pink">< center>
<table bgcolor="skyblue" border="2">< form method="post">< tr>
<td colspan=2 align="center">Employee Table</td></ tr>
<td>Name</td><td><input type="text" name="txt1"></td></ tr>
<tr>
<td>Designation</td><td><input type="text" name="txt2"></td></ tr>
<tr>
<td>Sal</td><td><input type="text" name="txt3"></td></ tr>
<tr>
<td>Qualification</td><td><input type="text" name="txt4"></td></ tr>
<tr>
<td><input type="submit" name="del" value="Delete"></td>< td><input type="submit" name="sel" value="Select"></td></ tr>
</form>
</table>
</center>
</body></ html Output
First of all you will select data from the database using the select button. When you will click on the select button then you will see the data table displayed on your web page. Like as in the following image.
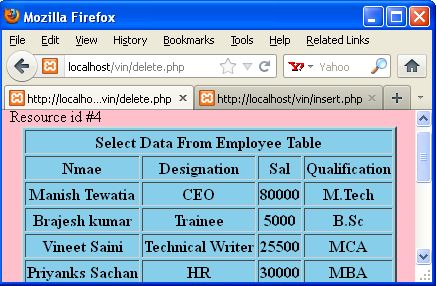
Suppose you want to delete Brajesh Kumar from your database. For this purpose you will fill in the name of the employee in the employee table, then click on the delete button. You can see in the following image.
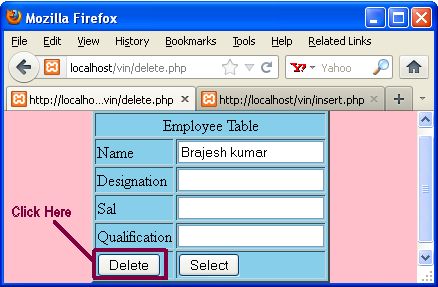
When you will click on the delete button then display a message on your webpage i.e. "Your Data Deleted".
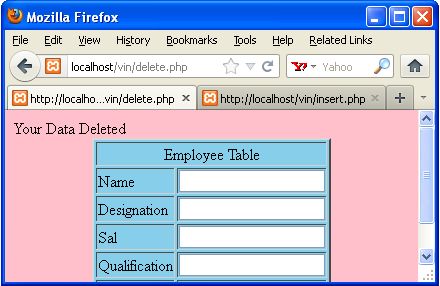
Now you will click on the select button for the selected data from the database. When you click on the select button then display data table on your web page. In this table you will see Brajesh kumar employee not exist. You can see in the following image.
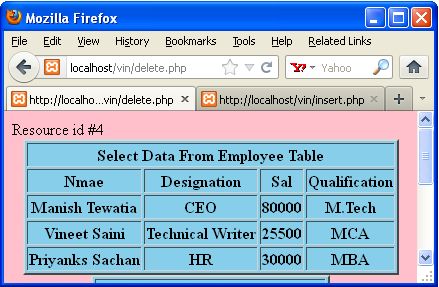
Conclusion
So in this article you saw how to delete data from a database in a HTML table (front end). Using this article one can easily understand how to delete data from a database.
Update Statement in PHP
In the previous article you saw how to insert and select data from a database to a HTML table. In this article you will see how to update data in a database through HTML table. Using the update statement you can update data in your database.
Code
$con=mysql_connect ("localhost","root","");
mysql_select_db ("mcn",$con);
@$a=$_POST['txt1'];
@$b=$_POST['txt2'];
@$c=$_POST['txt3'];
@$d=$_POST['txt4'];if(@$_POST ['up'])
{
$s="update employee set Designation='$b',Sal='$c',Qualification='$d' where Name='$a'";echo "Your Data Updated.";
mysql_query( $s);
}
$con=mysql_connect ("localhost","root","");
mysql_select_db("mcn",$con);if(@$_POST ['sel'])
{echo $ins=mysql_query ("select * from employee");echo "
Select Data From Employee Table | |||
---|---|---|---|
Nmae | Designation | Sal | Qualification |
while ($row=mysql_fetch_array($ins))
{
echo "
echo "
echo "
echo "
echo "
echo "";
}
}
echo ""
?><html>
<head>
</head>
<body bgcolor="pink"><center>
<table bgcolor="skyblue" border="2"><form method="post"><tr>
<td colspan=2 align="center">Employee Table</td></tr>
<td>Name</td><td><input type="text" name="txt1"></td></tr>
<tr>
<td>Designation</td><td><input type="text" name="txt2"></td></tr>
<tr>
<td>Sal</td><td><input type="text" name="txt3"></td></tr>
<tr>
<td>Qualification</td><td><input type="text" name="txt4"></td></tr>
<tr>
<td><input type="submit" name="up" value="Update"></td><td><input type="submit" name="sel" value="Select"></td></tr>
</form>
</table>
</center>
</body></html Output
First of all we select a table from the database like as in the following image. In the following table we will change the field of Manish Tewatia.
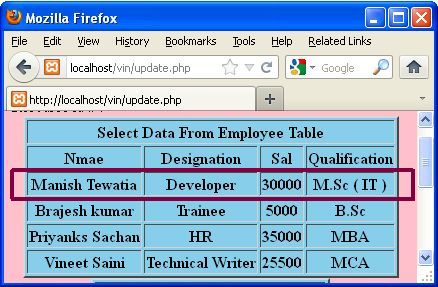
In the employee table we will fill the column for Manish Tewatia such as in the following image.
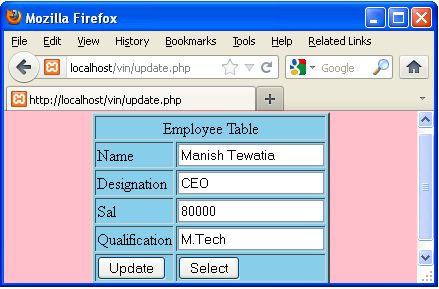
In the above table we filled designation, sal, qualification of Manish Tewatia. When you will click on the update button then your data will be updated.
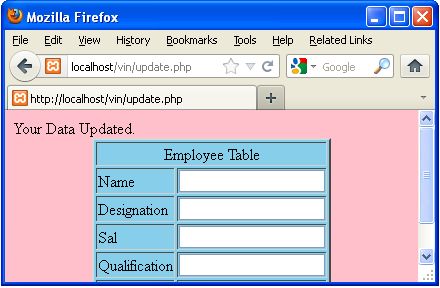
When you select a table from your database then you will get the update data of Manish Tewatia. Like as in the following image.
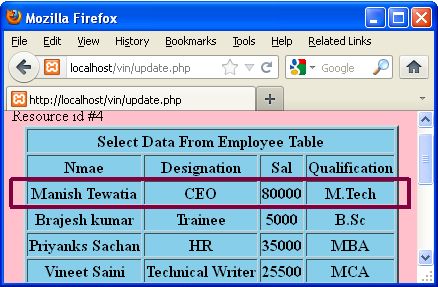
In the above table you will see, the designation, sal, qualification changed of Manish Tewatia.
Conclusion
So in this article you saw how to update data in the database. Using this article one can easily understand update statement in PHP.
Sorting Arrays in PHP
Introduction
Sorting is a process by which we can arrange the elements of a list in a specific order i.e. ascending or descending order. We can say sorting is the process of putting a list or a group of items in a specific order. Sorting may be alphabetical or numerical.
In PHP, sorting is a process of arranging the elements of an array in a specific order i.e. ascending or descending order. Using sorting you can analyze a list in a more effective way. You can sort an array by value, key or randomly. In the most situations we need to sort an array by value.
Some sorting functions are as follows:
- sort()
- asort()
- rsort()
- arsort()
Using the sort() function you can sort easily and simply. The example of the sort() function is as follows:
<body bgcolor="pink"><h3>Sort() Function</h3> $name=array ("Vineet","Arjun","Manish","Laxman");
echo "Before sorting the values are:".'
';
print_r($name).'
'.'
';
sort($name);
echo"
";
echo"
";
echo "After sorting values become:".'
';
print_r($name).'
';?></body>
In the above example we defined four elements (names) in the array. In this example we used the sort() function. The sort() function arranges the elements of an array in ascending (alphabetically) order. You can see in the following image:
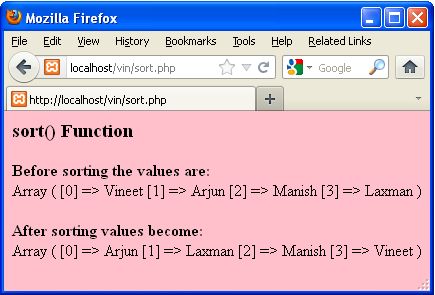
2. asort() function
In the example above you saw that the elements of the array are arranged in ascending (alphabetically) order. The asort() function sorts an array and also maintains the index position. An example of the asort() function is as follows:
<body bgcolor="pink"><h3>Sort() Function</h3> $name=array ("Vineet","Arjun","Manish","Laxman");
echo "Before sorting the values are:".'
';
print_r($name).'
'.'
'; asort($name);
echo"
";
echo"
";
echo "After sorting values become:".'
';
print_r($name).'
';?></body>
Output
In this output you will see the array sorted by their index position.
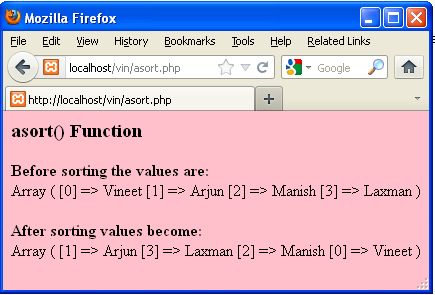
3. rsort() function
The rsort() function is used to sort an array in descending (alphabetic) order. An example of the rsort() function is as follows:
<body bgcolor="pink"><h3>Sort() Function</h3> $name=array ("Vineet","Arjun","Manish","Laxman");
echo "Before sorting the values are:".'
';
print_r($name).'
'.'
';
rsort($name);
echo"
";
echo"
";
echo "After sorting values become:".'
';
print_r($name).'
';?></body>
Output
In this output you will see the array sorted in a descending (alphabetically) order.
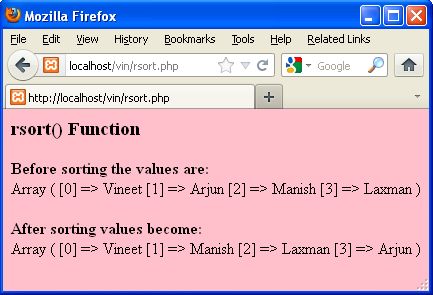
4. arsort() function
The arsort() function is a combination of asort() + rsort(). The arsort() function will sort an array in reverse order and maintain index position. The example of the arsort() function is as follows:
<body bgcolor="pink"><h3>Sort() Function</h3> $name=array ("Vineet","Arjun","Manish","Laxman");
echo "Before sorting the values are:".'
';
print_r($name).'
'.'
';
arsort($name);
echo"
";
echo"
";
echo "After sorting values become:".'
';
print_r($name).'
';?></body>
Output
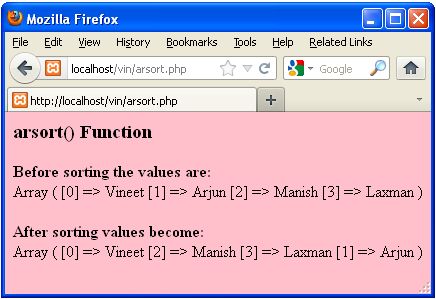
Conclusion
In this article you saw how to sort an array in PHP. Using this article one can easily understand sorting an arrays in PHP.
Database Connectivity (Insert and Select) With MySQL in PHP
This article explains how to insert and select data from a database into a HTML table in PHP. Using an insert statement you can insert data into a database. Using a select statement you can select the entire data from a database into a HTML table.
First of all we will create a database.
Create The Database
<html>
<body bgcolor="pink"><center>$con = mysql_connect ("localhost","root","");
$db="MCN";if (!$con)
{
die ('Could not connect: ' . mysql_error());
}if (mysql_query ("CREATE DATABASE $db",$con)) {
echo "Your Database Created Which Name is : $db";
}else
{
echo "Error creating database: " . mysql_error();
}
mysql_close ($con);?></center>
}</body></html>
Output
In the above code, we created a database named MCN. Now we will create a table in the MCN database.
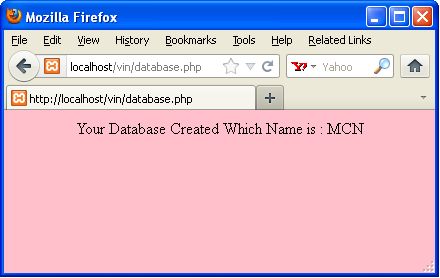
Create The Table
<body bgcolor="pink"><center>$con = mysql_connect ("localhost","root","");if (!$con)
{
die ('Could not connect: ' . mysql_error());
}
mysql_select_db ("MCN", $con);
$sql = "CREATE TABLE EMPLOYEE
(
Name varchar(50),
Designation varchar(50),
Sal int,
Qualification varchar(50)
)";
mysql_query($sql,$con);echo "Your table created which is as follows";
mysql_close($con);?><table bgcolor="skyblue" border="2"><tr>
<td colspan=2 align="center">Employee Table</td></tr>
<td>Name</td><td><input type="text" name="txt1"></td></tr>
<tr>
<td>Designation</td><td><input type="text" name="txt2"></td></tr>
<tr>
<td>Sal</td><td><input type="text" name="txt3"></td></tr>
<tr>
<td>Qualification</td><td><input type="text" name="txt4"></td></tr>
</table>
</center>
</body>
In the above code we created a table named employee. There are four fields in the employee table i.e. name, designation, sal, qualification.
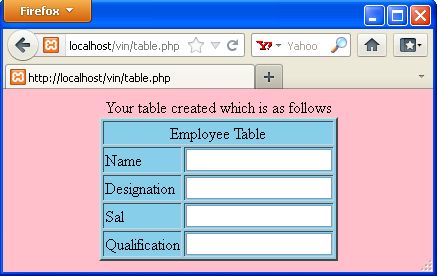
Insert Data in Table
Now we will write the code to insert data into the database table. The code for inserting data into the database table is as follows:
$con=mysql_connect ("localhost","root","");
mysql_select_db("mcn",$con);
@$a=$_POST['txt1'];
@$b=$_POST['txt2'];
@$c=$_POST['txt3'];
@$d=$_POST['txt4'];if(@$_POST['inser'])
{
$s="insert into employee values ('$a','$b','$c','$d')";echo "Your Data Inserted";
mysql_query ($s);
}
$con=mysql_connect ("localhost","root","");
mysql_select_db ("mcn",$con);if(@$_POST ['sel'])
{echo $ins=mysql_query ("select * from employee");echo "
Select Data From Employee Table | |||
---|---|---|---|
Nmae | Designation | Sal | Qualification |
while ($row=mysql_fetch_array ($ins))
{
echo "
echo "
";
echo "
echo "
echo "
echo "";
}
}
echo ""
?><html>
<head>
</head>
<body bgcolor="pink"><table bgcolor="skyblue" border="2"><form method="post"><tr>
<td colspan=2 align="center">Employee Table</td></tr>
<td>Name</td><td><input type="text" name="txt1"></td></tr>
<tr>
<td>Designation</td><td><input type="text" name="txt2"></td></tr>
<tr>
<td>Sal</td><td><input type="text" name="txt3"></td></tr>
<tr>
<td>Qualification</td><td><input type="text" name="txt4"></td></tr>
<tr>
<td><input type="submit" name="inser" value="Insert"></td><td><input type="submit" name="sel" value="Select"></td></tr>
</form>
</table>
</body></html Output
Now we will insert the data into a HTML table then click on the insert button.
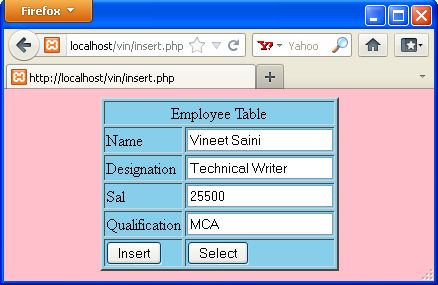
When you click on the insert button then your data will be inserted in the database table. Like as in the following image.
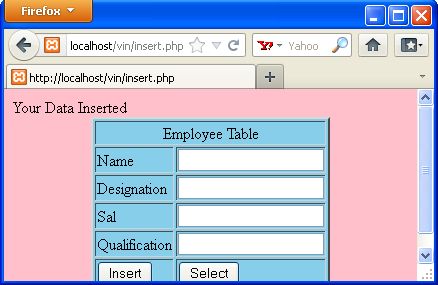
Select Data From Database
If you want to select all data from the database into the HTML table then you can do that. For this purpose you will be click on the select button. When you click on the select button then your data will be displayed in the HTML table, such as in the following image:
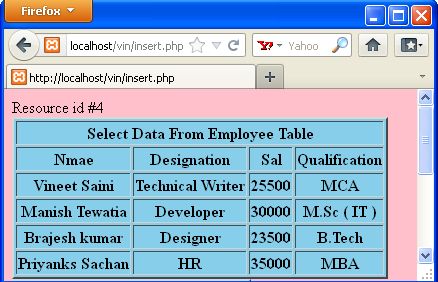
Conclusion
So in this article you saw, how to insert and select data from a database into a HTML table. Using this article one can easily understand insert and select data from database in to HTML table.
Subscribe to:
Posts (Atom)